program in C and C++ for half adder Half Adder Half adder is used for adding two bit.There are two input and two output in half adder. Truth Table: Circuit Diagram: Sum = A XOR B Carry = A AND B Program in C: #include <stdio.h> #include <conio.h> typedef char bit; bit carry=0; bit halfadd(bit A,bit B) { carry=A&B; return A^B; } int main() { int i,j,result; printf("A B | S Carry\n"); for(i=0;i<2;i++) { for(j=0;j<2;j++) { result=halfadd(i,j); printf("%d %d | ",i,j); printf("%d %d\n",result,carry); } } return 0; } Output: A B | S Carry 0 0 | 0 0 0 1 | 1 0 1 0 | 1 0 1 1 | 0 1 -------------------------------- Process exited after 0.1519 seconds with return value 0 Press any key to continue . . . Program in C++: #include <iostream> using namespace std; typedef char bit; bit halfadd(bit A,bit B,bi
Posts

program in C and C++ for half subtractor Half Subtractor Half subtractor is used to perform subtraction for two bit.There are two input and two output in half subtractor. Truth Table: Circuit Diagram: Diff = A XOR B Borrow = A' AND B Program in C: #include <stdio.h> typedef char bit; bit borrow=0; bit halfsub(bit A,bit B) { borrow=!A&B; return A^B; } int main() { int i,j,result; printf("A B | Diff Borrow\n"); for(i=0;i<2;i++) { for(j=0;j<2;j++) { result=halfsub(i,j); printf("%d %d | ",i,j); printf("%d %d\n",result,borrow); } } return 0; } Output: A B | Diff Borrow 0 0 | 0 0 0 1 | 1 1 1 0 | 1 0 1 1 | 0 0 -------------------------------- Process exited after 0.1232 seconds with return value 0 Press any key to continue . . . Program in C++: #include <iostream> using namespace std
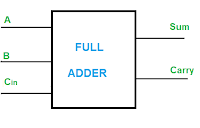
program in c and c++ for full Adder Full Adder Full Adder is a combinational logic circuit that perform the arithmetic sum of three inputs bits. There are three input and two output in Full adder. Truth Table: Circuit Diagram: Sum = A XOR B XOR C_in C_out = A.B + B.C_in + A.C_in Program in C: #include <stdio.h> typedef char bit; bit Cout=0; bit fulladd(bit A ,bit B,bit Cin) { Cout=(A&B)||(Cin&(A^B)); return (A^B)^Cin; } int main() { int i,j,k; int result; printf("A B Cin | S Cout\n"); for(i=0;i<2;i++) { for(j=0;j<2;j++) { for(k=0;k<2;k++) { result=fulladd(i,j,k); printf("%d %d %d | ",i,j,k); printf("%d %d\n",result,Cout); } } } return 0; } Output: A B Cin | S Cout 0 0 0 | 0 0 0 0 1 | 1 0 0 1 0 | 1 0 0 1 1 | 0 1 1 0 0 | 1 0 1 0 1 | 0 1 1 1 0

program in C and C++ for full subtractor Full Subtractor Full Subtractor is a combinational logic circuit that perform the arithmetic subtraction among three bits. There are three input and two output in Full adder. Truth Table: Circuit Diagram: Diff = A XOR B XOR B in B out = A'.B + B. B in + A'.B in Program in C: #include <stdio.h> typedef char bit; bit Bout=0; bit fullsub(bit A ,bit B,bit Bin) { Bout=(!A&Bin)||(!A&B)||(B&Bin); return (A^B)^Bin; } int main() { printf("A B Bin | Diff Bout\n"); for(int i=0;i<2;i++) { for(int j=0;j<2;j++) { for(int k=0;k<2;k++) { int result=fullsub(i,j,k); printf("%d %d %d | ",i,j,k); printf("%d %d\n",result,Bout); } } } return 0; } Output: A B Bin | Diff Bout 0 0 0 | 0 0 0 0 1 | 1 1 0 1 0 | 1 1 0 1 1 |

Program in C or C++ for JK flip-flop Program in c and c++ for JK flip-flop JK Flip-Flop JK flip-flop is capable of storing 1 bit. It has three inputs, labeled S(for set), R(for reset) and C(for clock). image source-google | image by- Wikimedia Commons Circuit Diagram: image source-google | image by- Wikimedia Commons Q(t+1)=J.Q(t)' + K'.Q(t) Program in C: #include <stdio.h> typedef char bit; bit jk(bit J,bit K,bit Q) { return J&~(Q)|Q&~(K); } int main() { int i,j,k,a,res=0; int x,y,z; char b; printf("1.Truth Table of JK flip flop"); printf("\n2.I/O operation\n"); printf("Enter your choice: "); scanf("%d",&a); switch(a) { case 1: printf("J K Q(t) | Q(t+1)\n"); for(i=0;i<2;i++) { for(j=0;j<2;j++) { for(k=0;k<2;k++) { res=jk(i,j,k); printf("%d %d %d |",i,j,k);

Program in C and C++ for RS flip-flop RS Flip-Flop RS flip-flop is capable of storing 1 bit. It has three inputs, labeled S(for set), R(for reset) and C(for clock). image source-google | image by- Wikimedia Commons Circuit Diagram: image source-google | image by- Wikimedia Common Q(t+1)=S + R'.Q(t) Program in C: #include <stdio.h> typedef char bit; bit rs(bit S,bit R,bit Q) { return (S)|(Q&(~(R))); } int main() { int i,j,k,a,res=0; int x,y,z; char b; printf("1.Truth Table of RS flip flop"); printf("\n2.I/O operation\n"); printf("Enter your choice: "); scanf("%d",&a); switch(a) { case 1: printf("S R Q(t) | Q(t+1)\n"); for(i=0;i<2;i++) { for(j=0;j<2;j++) { for(k=0;k<2;k++) { if(i==1&&j==1) { char r='x'; printf("%d %d %d ",i,j,k); printf(" | %c\n",r); } else